I tried using Coffeescript, looks like it was not made for me. So it is a language with a not so simple syntax that is supposed to compile back to JavaScript. The selling point is, if you come from a OOP background, it makes working with JavaScript easier. The catch is you need to know JavaScript first.
But this is not about coffeescript or the million other alternatives that compiles down to javascript. JavaScript has some reserved keywords that are not being used:
abstract class const extends implement package public private ...
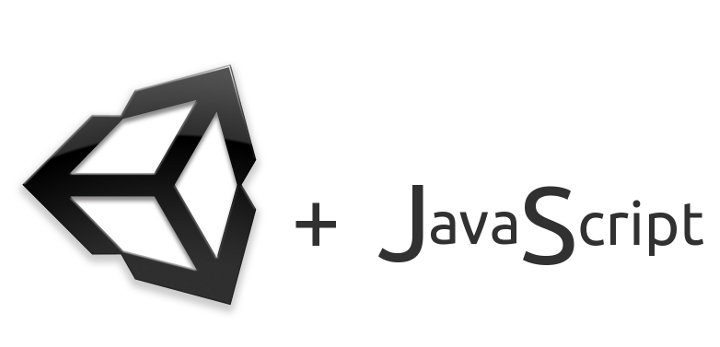
The reason we cannot implement those today is because they will break all legacy code, and most browsers won't support it. I recently started using the Unity 3D game engine and I was impressed with its implementation of JavaScript. It enforces type checking. That's all.
var health : int;
var timer : float;
var target : GameObject
function OnCollisionEnter(collider : Collision) {
var contact : ContactPoint = collision.contacts[0];
...
}
You get the idea. If we add this to JavaScript on the browser, all the code in the world will break. One thing that I appreciate in those languages that compiles into JavaScript, is that there is a compiler in the middle. JavaScript does not have a type system per se but the compiler can enforce it.
The class
keyword is not supported, but JavaScript does support classes in its own way. The compiler can simply replace the usual OOP class creation with JavaScript's own way.
class Cat extends Animal {
var lives: int = 9;
public function doCatThings(){
// ...
}
private function doEvilThings(){
// cats are evil when they are alone
}
}
var cat = new Cat();
The compiler first enforces all the types then convert it to whatever method is appropriate for creating a class:
// The result
function Cat() {
this.lives = 9;
this.doCatThings = function() {
// ...
};
var doEvilThings = function(){
// cats are evil in their private times
};
}
Cat.prototype = new Animal();
var cat = new Cat();
JavaScript developers do not need to learn yet another language that comes back down to JavaScript. Reusing these keywords that are already flagged as reserved words make much more sense.
The goal is to use a compiler that convert this simple syntax to the current implementations in JavaScript. This will give time for browsers to catch up and hopefully one day natively implement those keywords.
Comments
There are no comments added yet.
Let's hear your thoughts