In JavaScript, everything is an object. This is amazing because you never have to ask yourself if something is an object. The answer is always Yes. Let's look at a few examples:
// a number is an object
var num = 32;
num.toFixed(2);
> "32.00"
// a string is an object
var string = "hello world".replace("world","friend");
> "hello friend";
// Even a function is an object
function foo(){console.log("bar")}
foo.apply();
> "bar"
Everything can be treated as an object. Like any programming in JavaScript objects also inherit properties from parent objects. For example, when you create an array, you are automatically blessed with all the methods of the Array
object.
a = ['hello','world'];
a.join(" ");
> "hello world";
Join is a property found in the Array object. There are many more functions and properties and they are available to you anytime. However, even the Array objects inherit its properties from a parent object. And if you keep following them to the source, there is one object to rule them all. The Object.
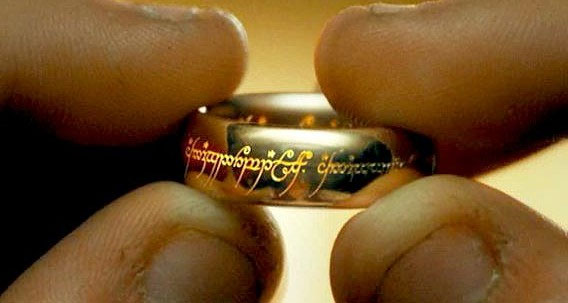
Every objects is a descendant of the Object.
Function instanceof Object > true
Array instanceof Object > true
String instanceof Object > true
...
So it becomes hard to differentiate objects because they all share many properties in common. If you want to set your own properties on an object, you may have trouble to determine if your property has really been set.
Let's take a few examples. Let's create an object that has the ability to be printed as a string.
var myObj = {
firstName: "Ibrahim",
lastName : "Diallo",
doWork : function(){
return "I work"
},
toText: function (){
return this.firstName+" "+this.lastName;
}
};
Now I want to be able to print this object in a string. Here is how I want to use it.
var content = "The author name is "+ myObj;
For it to properly work, I would have to change the function toText
to toString
. To make sure, I can check if the object has to has the toString
method. I can test it this way.
if (myObj.toString) // true
if ("toString" in myObj) // true
Both of these will return true
even though I haven't defined this function myself. The reason is, myObj
is a child of Object
, the one true object. When we try to verify that a property exists, the JavaScript engine goes through the entire object prototype chain to see if it exists always returning true. But to solve our problem, we want to check that the function exists only on the child object we created.
Object.hasOwnProperty
Luckily, the Object has a method just for that. And we can call it on any object basically. So here is how it works
myObj.hasOwnProperty("toString");
> false
This method doesn't go through the prototype chain, only the current object being tested.
Now we can go ahead and rename our method toText
to toString
if it fails the test. Using .hasOwnProperty
allows us to test only for properties we have manually created on the current object.
In most cases where we use the keyword in
as in “property” in Object, we can replace it with hasOwnProperty to make sure we are testing for stuff we created ourselves.
Sign up for the Newsletter.
Comments
There are no comments added yet.
Let's hear your thoughts