Parsing the browser User Agent is very unreliable. Not only users can easily manipulate it but the string can be very inconsistent. There were times where I needed to write specific code for Internet Explorer but I don't like writing in line JavaScript or loading a separate script just for that. I wish browser makers could agree on making unique GUID that can easily be looked up on a table to determine the browser. So Instead, I make use of IE conditional comments to set values that will only be present in Internet Explorer.
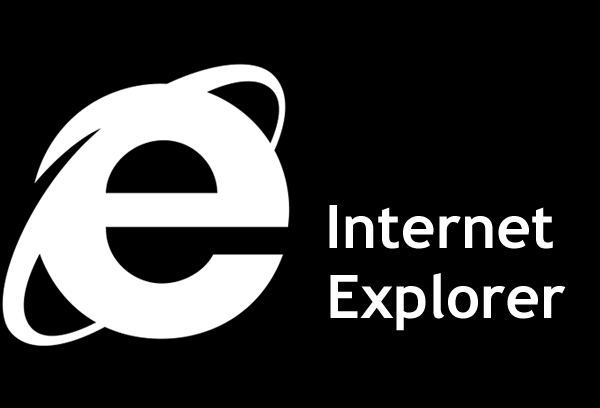
IE11 User Agent: Mozilla/5.0 (Windows NT 6.3; Trident/7.0; rv:11.0) like Gecko
Long ago I found a little trick on the web to detect if the current browser is IE. I don't remember and can't find the source but here is how it works:
var IE = (!+"\v1")?true:false; // true only in IE
I do not understand how this works internally, but all I know is that it works. Only IE will evaluate this statement to be true. I wouldn't really recommend using it because a couple days from now you will forget what it does.
Using conditional comments is nothing new. It was available in the earliest days of Internet Explorer. Here is a quick look at how it works:
<!--[if IE]>
Hello World
<![endif]-->
All browsers other than IE will see this as an HTML comment and will ignore it, <!-- This is an html comment -->
. This can used to pass some code that only Internet explorer will read. It can also be used to target a specific version of the browser:
<!--[if IE 6]>I am Internet Explorer 6<![endif]-->
<!--[if IE 7]>I am Internet Explorer 7<![endif]-->
So we can use this feature to set a JavaScript global to know which version of the browser we are running.
<script> var Browser = {isIE:false};</script><!-- By default we will assume that the browser is not IE -->
<!--[if IE 5]><script>Browser = {isIE:true,version:5};</script><![endif]-->
<!--[if IE 6]><script>Browser = {isIE:true,version:6};</script><![endif]-->
<!--[if IE 7]><script>Browser = {isIE:true,version:7};</script><![endif]-->
<!--[if IE 8]><script>Browser = {isIE:true,version:8};</script><![endif]-->
<!--[if IE 9]><script>Browser = {isIE:true,version:9};</script><![endif]-->
<script type="text/javascript" src="path/to/your/script.js"></script>
The Browser
object is now available in your external scripts and if the user is using Internet Explorer you will be aware of it.
// External js file
if (Browser.isIE){
if (Browser.version < 8){
// Commence attack.
var user = new Target(),
drone = GetClosestDrone();
user.setInfo(GetUserIp(),GetUserGPS());
drone.setTarget(user);
drone.destroy();
while(user.status == 200){
drone.destroy();
}
}
}
This code will only run if the browser is IE and have a version lower than 8. Writing code for a specific browser is not the recommended approach, it is better to detect if features are available instead. Example:
// cross browser event handling
var obj = document.createElement("a"),
addEvent = obj.addEventListener? 'addEventListener': 'attachEvent',
removeEvent = obj.removeEventListener? 'removeEventListener':'detachEvent',
on = obj.addEventListener? '':'on';
var button = document.getElementById("button");
button[addEvent](on+"click",function(){doAmazingThings();},false);
This will work on browsers that support addEventListener
or attachEvent
. However there are times where you actually need to know what browser is running. For example you want to track how many of your users are running IE 7. In this case you would use the browser detect method describe in this article.
July 2015 Update: Here is how you may break the web if you use browser detection
Comments(2)
Jonas :
Lol I love the code to destroy less than IE8 users. It would have been better if you destroy IE all together
Jerry :
IE9 supports addEventListener.
Let's hear your thoughts